Baseball Scoreboard
When watching Henry Blevins' YouTube video about React Testing with React Testing Library and Jest (which I wrote about in a previous post), he uses a simplified baseball scoreboard as part of the exercise. I wanted to flesh it out more.
👉 View the baseball scoreboard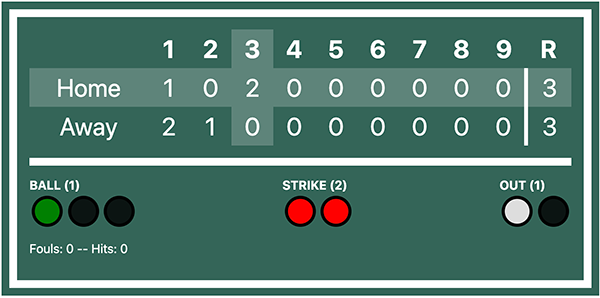
To use it, click the button controls under the display. Though Henry's video touches on some minor logic functions (clicking 'hit' should clear out any existing balls, strikes, or fouls), I got to add a few more of my own, like advancing innings when three outs were reached.
I also got exposed to a few more items.
First, I was no longer defining state as just a string, boolean, or number. This time, in order to keep track of the score over each inning, I needed to define the state using an object. This meant I then needed to set state for just one key / value pair of that object. One does so by spreading the object, then specifying which key value pair to overwrite:
setRuns((prevRuns) => ({
...runs,
[`inning${currentInning}${currentTeam}Runs`]:
prevRuns[`inning${currentInning}${currentTeam}Runs`] + 1,
}));
Second, styling. I didn't want this to be a styling exercise, so I wanted to purposefully leave out styling dependencies such as Sass, Tailwind, or styled-components. So I just went with styles within the jsx itself:
<style jsx>{`
.display {
background: #346558;
border: 10px solid #fff;
padding: 1em;
outline: 10px solid #346558;
margin: 2em;
color: #fff;
display: inline-block;
}
...
</style>
Third, I ran into issues during the build that pointed out that I could not have a components
directory within the pages
directory. I was hoping to keep my page-specific components co-located with the page I was working on, and though this worked fine during local development, it did not work well for the build. The fix is to move components outside of the pages
directory.
Overall this was a fun exercise in styling, using state, and separation of files and functions.